In our modern, high-speed world, web application users expect rapid load times and smooth, effortless navigation. React is a widely used front-end library, powering approximately 11,908,579 live websites, and is renowned for its exceptional performance.
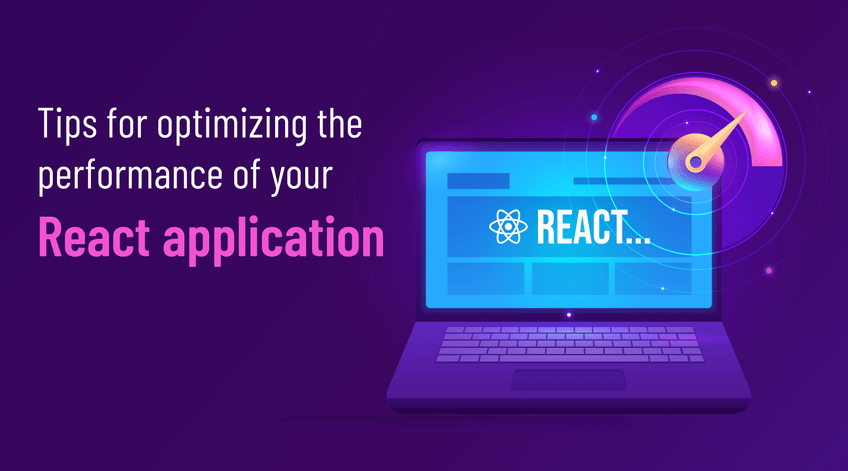
However, ensuring its continued high performance is crucial. If your React application takes an eternity to load or feels unresponsive during use, it's unlikely that users will remain engaged for long.
Optimizing the performance of your React Native application is key to providing a seamless user experience and keeping users engaged. But where do you start?
No need to worry, we've got you covered.
In this article, we'll dive into the top tips to improve React Performance. From measuring performance regularly to using lazy loading and code splitting, we'll provide actionable advice to help you take your React Native application to the next level and hire react native developers based on these tips.
So, let's get started on optimizing your React Native application for peak performance.
Measure Performance
Measuring performance is the first step towards optimizing it. How do you know if your React application is fast enough unless you measure its performance regularly?
Fortunately, measuring performance in React is easier than you might think. There are several tools available to help you understand how your application is performing, the most popular react native tools include the React Profiler and Lighthouse.
The React Profiler is an integrated tool for measuring the performance of your React components. It provides detailed information about which components are taking the longest to render, allowing you to focus your optimization efforts on where they'll have the biggest impact.
And the best part? There is no need to install any additional tools because it is built into React.
Lighthouse is another great tool for measuring the performance of your React application. It's a Google open-source project that analyzes your application's performance, accessibility, best practices, and other factors.
Simply run Lighthouse on your application and it will give you a report highlighting areas for improvement, along with actionable advice to optimize performance.
Measuring performance regularly is key to ensuring your React application stays fast and responsive. By using the React Profiler and Lighthouse, you can get a clear picture of your application's performance and focus your optimization efforts where they'll have the biggest impact.
Use lazy loading and code splitting
Lazy loading and code splitting are two performance optimization techniques that can have a big impact on the speed and responsiveness of your React mobile application.
You can ensure that only the code required to render a specific component is loaded by using lazy loading and code splitting, reducing the overall size of your application and improving load times.
Lazy loading is the process of deferring the loading of certain components or assets until they're actually needed. If your application contains a large number of images, you can lazy load them so that they only load when the user scrolls to the section of the page where they are displayed.
This can greatly reduce the initial load time of your application and improve overall performance.
Code splitting is similar to lazy loading, but it refers to splitting your application's code into smaller chunks that can be loaded on demand. It allows you to optimize the size of your application and reduce load times.
For example, you might split your application's code into separate chunks for different routes or sections of your application. When a user navigates to a specific route, only that route's code is loaded, improving the performance of your application.
Minimize the number of components
Less is often more when it comes to optimizing the performance of your React application. This is especially true if your application contains a large number of components.
The more components you use, the more work React has to do to render and update your app, which can result in slower load times and a less responsive user experience.
Reducing the number of components in your application is a good way to boost its performance. This can be done in a variety of ways, including using higher-order components, functional components, and React.memo() function.
Higher-order components are components that wrap other components to add additional functionality. You can reduce the number of components in your application and simplify its structure by using higher-order components.
Functional components are components that are defined as functions, rather than classes. They're lightweight and simple, and they can often be used in place of class components to reduce the number of components in your application.
The React.memo() function is another great tool for minimizing the number of components in your application. It allows you to memoize a component, which means that React will only re-render the component if its props have changed.
This can greatly improve the performance of your application, especially when used in combination with functional components.
Avoid expensive computations
Expensive computations can be a real performance killer in your React application. When your components are performing a lot of work, they can cause your application to slow down and feel less responsive.
To avoid this, it's important to be mindful of the computations your components are performing and to minimize their complexity.
There are a few ways to minimize the complexity of your computations in React.
-
One approach is to use the useMemo() hook, which allows you to memoize expensive computations so that they're only performed when necessary. If you have a component that performs a complex calculation, you can use the useMemo() hook to memoize the result of that calculation so that it is only performed once, even if the component re-renders multiple times.
-
Another approach is to use the useCallback() hook, which is similar to useMemo() but is specifically designed for memoizing callback functions. If you have a component that re-renders frequently, you can use the useCallback() hook to memoize its callback functions so that they're only created when necessary, reducing the amount of work your component has to do.
-
When working with arrays and objects, it's critical to be aware of the operations you're performing in your components. For instance, it's often more efficient to use the map() function to transform arrays than it is to use for loops. Similarly, it's more efficient to use the object spread operator than it is to use Object.assign().
Use memoization
Memoization is a technique that can help you optimize the performance of your React application by avoiding unnecessary calculations and rendering. Memoization, in a nutshell, allows you to cache the results of expensive computations and return them whenever possible, rather than recalculating the results every time.
React provides several built-in tools for memoization, including the useMemo() and useCallback() hooks. These hooks allow you to memoize values and functions, respectively, so that they're only recalculated when necessary.
If you have a component that performs a complex calculation, for example, you can use the useMemo() hook to memoize the result of that calculation so that it is only performed once, even if the component re-renders multiple times.
Another useful tool for memoization in React is the React.memo() function, which allows you to memoize functional components. When you wrap a functional component in React.memo(), React will only re-render the component if its props have changed, improving the performance of your application.
It's worth noting that memoization is a trade-off between performance and memory usage. By memoizing values and functions, you're using up more memory to store the cached results, but you're also reducing the amount of work your application has to do.
As such, it's important to be mindful of the values and functions you're memoizing and to balance performance and memory usage to find the right balance for your application.
Read more: Best React Native developer tools to create high-performing app
Use efficient algorithms
Using efficient algorithms is a key part of optimizing the performance of your React application. Whether you're working with data structures, searching through arrays, or sorting elements, it's important to use algorithms that are optimized for performance so that your application stays fast and responsive.
To enhance the performance of your React application, using efficient algorithms is crucial. Whether manipulating data structures, searching arrays, or sorting elements, choose algorithms optimized for speed to maintain a responsive and fast app.
When selecting algorithms, time complexity should be a top consideration. Time complexity describes the time an algorithm takes to complete based on the input data size.
For instance, linear search has a time complexity of O(n), meaning it takes longer to complete as data size increases. Binary search, on the other hand, has a time complexity of O(log n), becoming faster as data size increases.
Space complexity, the memory an algorithm needs to complete, is also important to consider. Bubble sort has a space complexity of O(1), constant memory use regardless of data size, while quicksort has a space complexity of O(log n), requiring more memory with increasing data size.
Finding the right balance between time and space complexity is vital when choosing algorithms for your React application. For instance, a binary search with O(log n) time and space complexity is ideal for large data and quick searching.
Conversely, bubble sort with O(n^2) time and O(1) space complexity is suitable for limited memory and in-place sorting.
Conclusion
Optimizing the performance of your React application is an important task that requires careful consideration and a thorough understanding of best practices.
By measuring performance, using lazy loading and code splitting, minimizing the number of components, avoiding expensive computations, using memoization, and choosing efficient algorithms, you can significantly improve the speed and responsiveness of your application.
With these pointers in mind, you'll be well on your way to developing a high-performance React app that provides a pleasant and satisfying user experience.
If you are looking to hire dedicated React Native developers, ThinkODC is the right choice. At ThinkODC, you'll get skilled and experienced React Native developers who can fulfill all your react development needs.