Fitness tracking applications have exploded in popularity in recent years, allowing users to monitor and take control of their health and fitness journeys. With these apps, people can set goals, log activities, record workouts, track calories burned, and more all from the convenience of their smartphones.
This rise in demand for fitness apps is evidenced by the rapid growth of the market. As per a report by Grand View Research, the global fitness app market size was valued at USD 1.3 billion in 2022 and is expected to expand at a CAGR of 17.6% from 2023 to 2030.
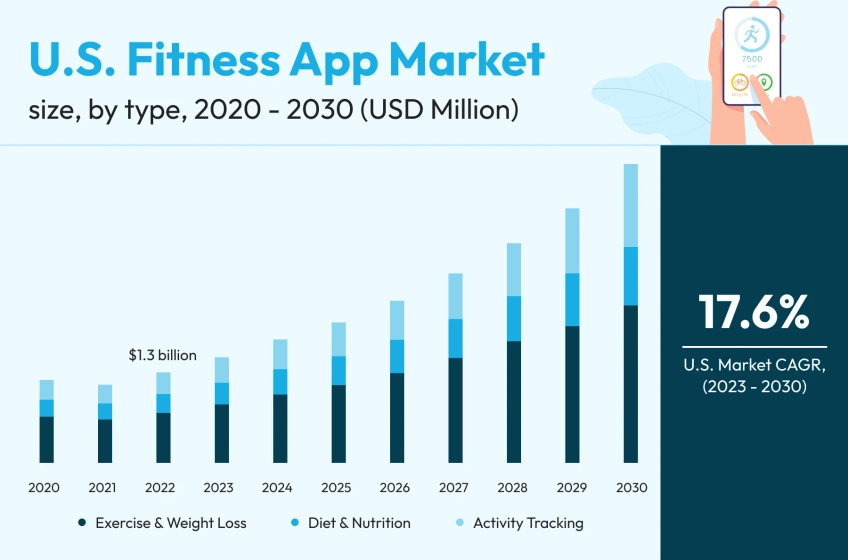
This tremendous growth underscores the rising adoption of fitness apps across the globe as more people seek to improve their well-being. The popularity of wearable devices like smartwatches and fitness bands that sync with smartphone apps has further fueled the fitness app craze.
So, at this time, developing your own fitness-tracking application can be an exciting endeavor, but choosing the right framework is crucial. Flutter has emerged as one of the top options for building cross-platform mobile apps.
In this guide, we'll walk through the entire process of developing a fitness-tracking mobile application using Flutter while optimizing for search engine visibility.
Understanding Fitness Tracking Applications
Fitness-tracking applications are software programs designed to monitor and track fitness activities, biometrics, and overall health progress. The main goal of these apps is to enable users to take control of their fitness journey.
Some top features of fitness apps include:
Activity Tracking: Using sensors and input from users, these apps track steps taken, distance covered, calories burned, heart rate, sleep cycles and more.
-
Goal Setting: Users can set goals related to steps, distance, exercise time or weight loss and track their progress.
-
Data Visualization: Charts, graphs and reports help users make sense of all the tracked data over time to spot trends.
-
Social Sharing and Competition: Users can share their achievements with friends and family and participate in challenges.
-
Reminders and Notifications: To motivate users and help build fitness habits.
As fitness-tracking apps provide valuable insight into one's health, they have become highly desirable both for users and developers.
Now that you have a basic understanding of fitness tracking applications, let us explore why you should choose Flutter for creating one.
Why Choose Flutter for App Development
Flutter is an open-source UI framework developed by Google for creating cross-platform mobile applications. Here are some key reasons to choose Flutter for a fitness app:
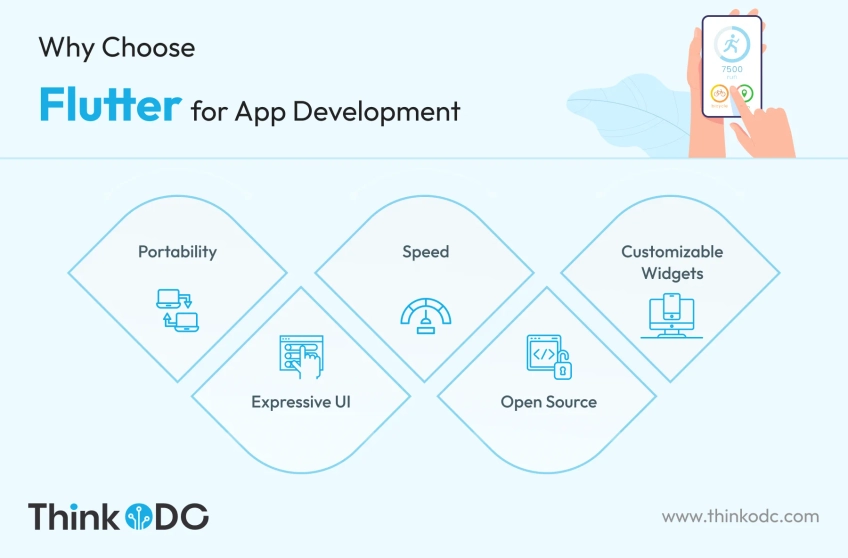
-
Portability- Flutter apps can be compiled to run natively on both iOS and Android from a single codebase. This eliminates the need to build apps separately for each platform.
-
Expressive UI- Flutter's layered architecture and reactive widgets make it easy to create beautiful native-like interfaces. Transitions and animations can be easily implemented.
-
Speed- Flutter's JIT compiler and functional reactive programming model result in fast rendering and rapid development cycles. Apps can be quickly built and tested.
-
Open Source- Flutter is entirely free and open-source with a supportive community. There are numerous learning and development resources available.
-
Customizable Widgets- Flutter provides a diverse catalog of pre-built widgets that can be customized extensively to craft unique interfaces.
For fitness tracking applications, Flutter can help build an intuitive and responsive user experience across platforms quickly and efficiently.
Read more: Xamarin vs. Flutter: Choosing the Best Cross-Platform Framework
Preparing for Development
Jumping straight into app development without sufficient planning often leads to failure. Here are some recommendations for preparing for fitness tracker app development:
-
Identify Target Audience- Determine who your app is meant for - fitness enthusiasts, casual exercisers, senior citizens etc. This affects design and feature decisions.
-
Analyze Competition- Research similar apps, the features they offer, their monetization strategies and reviews by users. This gives insights into standing out.
-
Define Goals- Decide on the primary purpose of your app - is it increasing user activity levels or promoting healthy habits? Establish success metrics.
-
Plan Features- Outline must-have features, nice-to-have additions and potential future enhancements. Prioritize critical features.
-
Choose Tools and Services- Figure out development tools, third-party services for the backend, payments etc. early for easy integration later.
-
Conceptualize UI/UX- Map out an effective yet intuitive user flow. Maintaining simplicity while meeting all user needs is important.
With thorough planning and research, you can build a unique value proposition for your fitness tracker app.
Setting Up Flutter Development Environment
Now we are ready to set up Flutter and start building the fitness tracker app. Here are the steps:
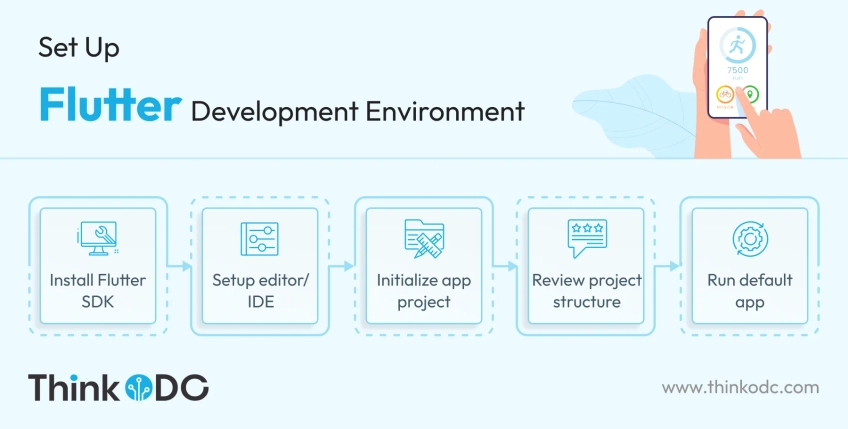
-
Install Flutter SDK: Go to https://flutter.dev/docs/get-started/install to install Flutter on your OS like Windows, macOS or Linux.
-
Setup editor/IDE: You can code Flutter apps in editors like Visual Studio Code or Android Studio. Install any of these and the required plugins.
-
Initialize app project: Open the command prompt/terminal to initialize your Flutter project using the "flutter create" command.
-
Review project structure: The initialized project contains directories like /lib for code, /test for tests, /assets for static files etc. Familiarize yourself with the structure.
-
Run default app: Enter "flutter run" in the terminal to compile and run the default Flutter app on a simulator or physical device. Verify Flutter is set up properly.
With Flutter installed, an IDE configured and a starter app running smoothly, you can now begin app development. Use Git for version control.
Creating User Interface with Flutter Widgets
Flutter's declarative UI development style makes it simple to create interactive user interfaces. Here are the steps to design the fitness tracker app's UI:
-
Install required packages: Add necessary packages like google_fonts, flutter_icons etc using the "flutter pub add" command.
-
Create reusable widgets: Components like buttons, navigation drawer, and input fields can be extracted into reusable widgets for easy maintenance.
-
Implement app theme: Use the ThemeData class to define consistent styling, colors and typography across the app. Apply custom themes to specific widgets.
-
Build UI for key screens: Use Flutter's powerful widget set to design visual layouts for app signup, activity dashboard, running tracker and other screens. Apply best practices like responsive design.
-
Add animations: Use Flutter's animation framework or packages like Flare to add subtle microinteractions. This enhances user delight.
-
Connect app navigation: Implement routes and navigator to enable navigation between screens using material app class properties.
With its extensive widget set and composable architecture, Flutter enables building fitness app UIs that are welcoming, intuitive and easy to navigate.
Implementing Core Fitness Tracking App Features
Now we are ready to code up the main app functionality using Dart and Flutter frameworks:
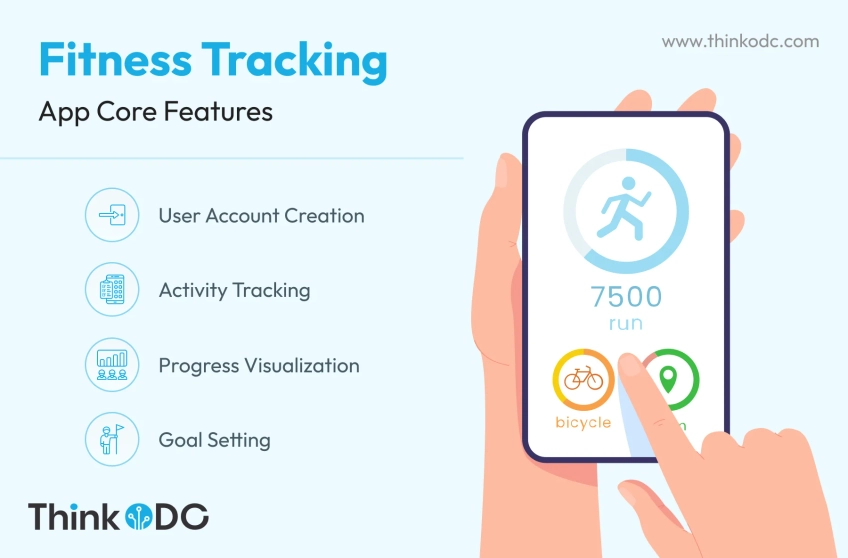
User Account Creation
- Use Firebase Authentication to enable easy email/password signup and login.
- Allow linking social media accounts like Facebook/Google for quicker onboarding.
- Store user profiles on Firestore/MongoDB database.
Activity Tracking
- Use pedometer and accelerometer sensors to track steps, distance, calories.
- Allow manual entry of workouts like cycling, weightlifting etc.
- Use Google Maps SDK to record location during walks, runs and hikes.
Progress Visualization
- Integrate time-series charts using Syncfusion/Echarts to visualize daily, weekly and monthly trends.
- Display simple summary cards of total steps, distance and calories.
- Generate detailed analytics reports with filtering for easy insights.
Goal Setting
- Allow input of custom goals like target weight, steps per day or running distance goals.
- Track progress towards each goal using analytics and time series graphs.
- Send notifications when goals are met or milestones are achieved to motivate users.
Integrating Backend and Data Management
Robust data management and integration with backend services are crucial for fitness apps dealing with user data. Here are some tips for the backend:
- Evaluate backend requirements for factors like app scale, complexity, costs to develop a fitness mobile app etc.
- Choose a BaaS like Firebase/Supabase for simplicity or build a custom backend with Node.js, Django etc.
- Define database schema for collections like Users, Activities, Goals etc. Use MongoDB, Postgres etc.
- Implement APIs for CRUD operations allowing the app frontend to seamlessly manage data.
- Utilize cloud services like AWS, and GCP for hosting backend application servers and databases.
- Ensure robust security measures like hashing passwords, encrypted data storage, HTTPS etc.
- Leverage Flutter packages like HTTP for API calls from the app and transmitting data.
For a flawless fitness app experience, optimize the backend to provide fast and secure data operations.
Rigorous Testing and Quality Assurance
To deliver a polished, high-quality fitness tracking app, extensive testing is crucial. Here are some key testing strategies:
-
Unit Testing- Isolate and test individual functions, classes and components using Dart's unit test framework.
-
Widget Testing- Flutter provides widget testing support to quickly pinpoint UI issues.
-
Integration Testing- Verify that components work together correctly across different OS versions and devices.
-
User Acceptance Testing- Conduct UAT with real users across different use cases to identify gaps.
-
Automated Testing- Use CI/CD pipelines to run automated regression tests every time new code is committed.
-
Beta Testing- Release beta versions for groups of real users to use under varied conditions before launch.
Prioritizing testing will help avoid bugs and deliver the best user experience. Monitoring app performance and stability post-launch is also recommended.
Optimizing Performance
Performance is a huge factor in user experience. Here are some performance optimization tips:
- Minimize app size by removing unused resources, enabling code shrinking, optimizing images etc.
- Debug using DevTools to identify bottlenecks like slow build times, excessive RAM usage etc.
- Analyze using Dart profilers to pinpoint resource-intensive code. Optimize identified code blocks.
- Implement infinite listviews to render only visible widgets instead of all data.
- Make API calls asynchronous to prevent UI freezes. Show progress indicators during network requests.
- Follow best practices like using const constructors, extracting heavy computations from build methods etc.
- Test on both iOS and Android to ensure consistency in speed and responsiveness.
With Flutter's native compilation, you get high performance by default. Additional optimizations will further enhance the app experience.
Deployment and App Store Presence
Once the app is thoroughly tested, you can deploy it to app stores:
- Enroll in the Apple Developer Program and Google Play Console for publishing apps.
- Ensure the app adheres to all platform policies around content, privacy, trademarks etc.
- Create listings highlighting app features. Include descriptive titles, quality icons, screenshots etc.
- Assign relevant keywords targeting your app's domain for better App Store SEO.
- Release beta versions for user feedback. Address bugs and gauge demand.
- Submit the app for review and publish updates to reach wider audiences.
- Monitor ratings and keep improving the app with user feedback. Respond promptly.
Following app store best practices will make your fitness app easier to find and download for relevant users.
In summary, developing fitness apps with Flutter helps tap into the thriving mobile health domain. Flutter's cross-platform abilities, rich UI widgets and modern tooling simplify building feature-packed fitness trackers.
Use the actionable guide provided to architect, code and deploy your own successful workout-tracking app. Integrate cutting-edge features and keep improving to help users achieve their health goals efficiently.
Looking to build your next mobile app with Flutter?
Get it done affordably by hiring dedicated mobile app developers from ThinkODC. Our experts have years of experience building high-performing cross-platform apps with Flutter. They follow agile practices for faster delivery.
With flexible engagement models, proven expertise, and low costs, ThinkODC is your ideal partner for hiring offshore Flutter developers. Partner with ThinkODC, a mobile app development company today for app success!
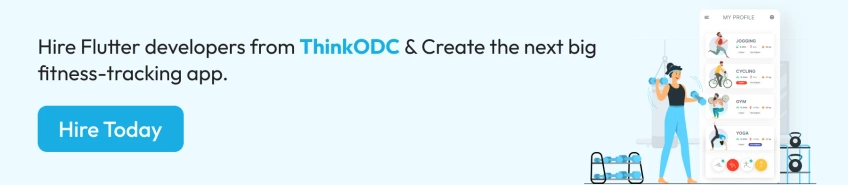